If you want to become a better JavaScript programmer, mastering JavaScript variable declarations is a great first step.
This article will explain the different types of declarations, as well as how and when to use them in order to optimize your code and improve performance.
What is JavaScript Variable Declaration?
JavaScript variable declaration is the process of telling a program what type of data will be stored in a reserved memory location. Variables are created at runtime and use two keywords: let and const. When applied to variables, the keyword let allows for the value of the variable to change at any point, whereas const creates a read-only variable that cannot be modified after it is declared. Both are helpful for organizing code and making debugging easier.
Different Types of JavaScript Variables
The two main types of variables in JavaScript are primitive and non-primitive.
Primitive variables include numbers, strings, booleans, and special values (null, undefined), while non-primitive variables include objects, arrays, and functions.
Variables declared with let can be used for both primitive and non-primitive types. Variables declared with const must have a value assigned to them when they are declared and that value must be primitives. Non-primitives cannot be assigned using const due to their dynamic state.
Var vs. Let vs. Const – When to Use Which
When writing code, JavaScript variables should always be declared with the appropriate declaration keyword. It’s important to understand the difference between var, let, and const so that you can determine which is most appropriate in any given situation.
Var should be used when declaring a variable that could be reassigned or updated at a later time.
Let should be used when declaring a variable that will only ever need to hold one value or is unlikely to ever change over the course of code execution.
Const should be used when declaring a variable that will always remain constant and never change throughout code execution.
Declaring a Variable
Declaring a variable in JavaScript is simple. Simply write the keyword followed by the name of your variable, then set it equal to a value.
For example, if you wanted to store the value “5” in a variable named “num”, you would use a statement like this:
var num = 5;
The statements below could be used to declare variables with let and const as well.
let num = 5;
const num = 5;
Understanding Scope Rules and Variable Lifecycle
In addition to understanding the three types of variable declaration in JavaScript, it is important to understand the scope rules and lifecycle of variables. A variable’s scope determines where it can be accessed from, while its lifecycle determines how long it remains accessible.
The two main scopes are global and local, with global variables being available from anywhere within the program, while local variables only exist within a particular section of code. The variable’s lifecycle is determined by when it is declared and how far down in the code block its value persists or changes.
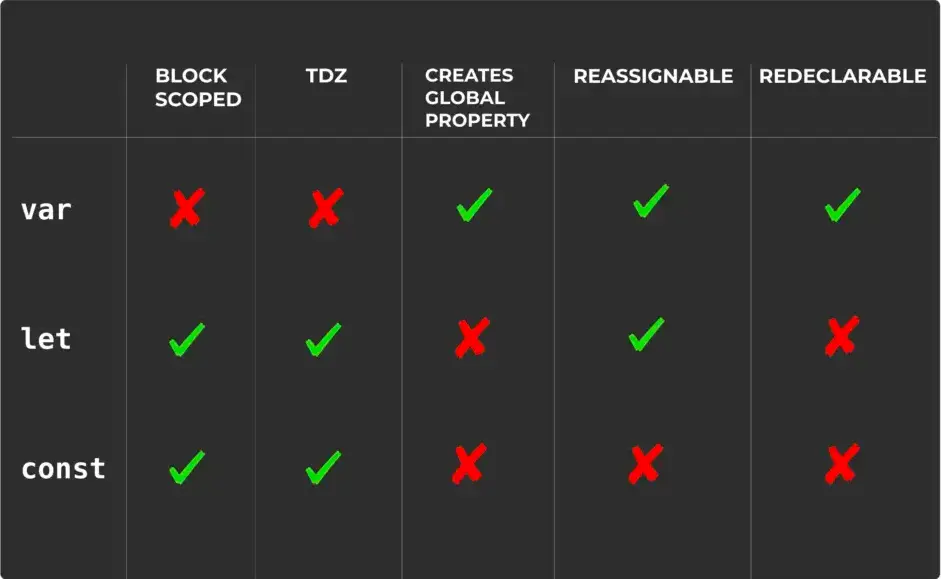
Leave a Reply