A JavaScript console is a powerful tool that can be used to debug and troubleshoot web pages. It enables us to execute JavaScript expressions in the contents of the page, which can be extremely useful for debugging purposes. In this article, we will take a look at some of the features of the Web console and how it can be used to debug web pages.
console.log()
console.log() is a function in JavaScript used to print the output to the javascript console. It can take any type as an input, be it a string, array, object, boolean, etc. The simplest use of this function is to log(print) the output to the console. We can also use it to debug our code by printing intermediate values to see how our program is running.
console.log('abc');
console.log(1);
console.log(true);
console.log(null);
console.log(undefined);
console.log([1, 2, 3, 4]); // array inside log
console.log({a:1, b:2, c:3}); // object inside log
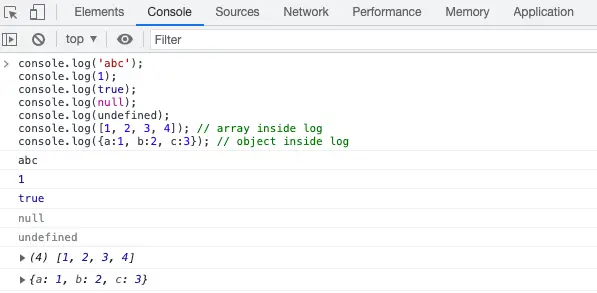
console.error()
The console.error() method is used to log error messages to the javascript console. This is useful in the testing of code, as it allows you to easily find and fix errors. The default behavior is to highlight the error message in red color.
// console.error() method
console.error('This is a simple error');

console.warn()
console.warn() is used to log warning messages to the javascript console. By default, the warning message will be highlighted with yellow color. The goal of this function is to provide a way for developers to easily find and fix potential problems in their code.
//console.warn() method
console.warn('This is a warning.');

console.clear()
console.clear() is a function that is used to clear javascript console. The console will be cleared, in this case a simple overlayed text will be printed like: ‘Console was cleared’. This function can be extremely useful if you want to make sure that your code is running properly and that there are no errors being displayed in the console.
// console.clear() method
console.clear();

console.time() & console.timeEnd()
The console.time() method starts a timer with a name specified as an input parameter and records the amount of time spent by the code block till console.timeEnd() is called with the specified name. If we want to know how long any given operation takes in JavaScript, this technique can come in handy. The performance measurement features provided by most browsers nowadays allow us to get more precise timings, but those are usually used to measure the whole page load time or specific components’ initialization times. console.time(), on the other hand, provides us with a way to measure just about anything we want inside our code.
// console.time() and console.timeEnd() method
console.time('abc');
let fun= function(){
console.log('fun is running');
}
let fun2= function(){
console.log('fun2 is running..');
}
fun(); // calling fun();
fun2(); // calling fun2();
console.timeEnd('abc');
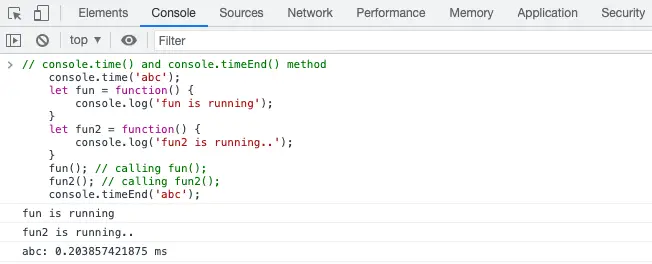
console.table()
The console.table() method is a powerful way to log data in tabular format. This can be especially useful when debugging or working with large datasets. The method takes an array or object as input and outputs a table in the console. The table will include all of the object’s properties and their corresponding values.
// console.table() method
// Object
console.table({'a':1, 'b':2});
// Array
console.table([{'a':1, 'b':2},{'a':2, 'b':3}]);
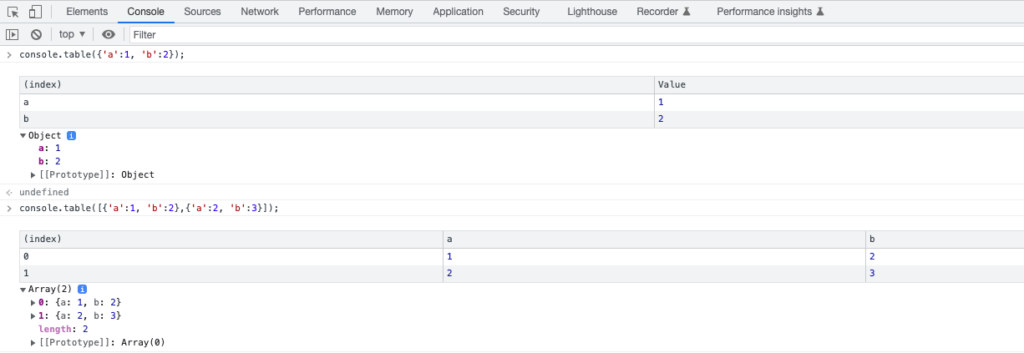
console.count() & console.countReset()
The console.count() method is a useful way to keep track of the number of times a particular callback function has been called. This can be helpful when debugging code, or simply when trying to understand the flow of execution in a complex program. If you’ve been using the console.count() method to keep track of how many times something happens on your website, you can use the console.countReset() method to reset the counter.
// console.count() method
for(let i=0;i<5;i++){
console.count(i);
};
console.countReset()
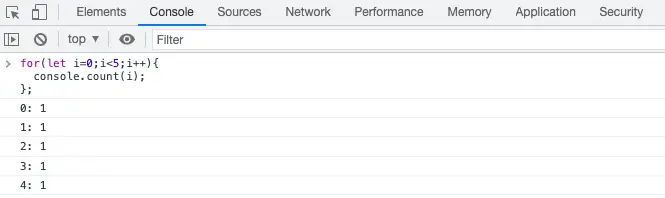
console.group() & console.groupEnd()
The console.group() and console.groupEnd() methods of the console object are used to group together related output in a separate block that is indented. Both methods accept an optional label argument, which can be any value that can be coerced to a string. The label is used to identify the group in the output.
console.group('w3tweaks');
console.warn('warning!');
console.error('error here');
console.log('log w3tweaks');
console.groupEnd('w3tweaks');
console.log('new section');
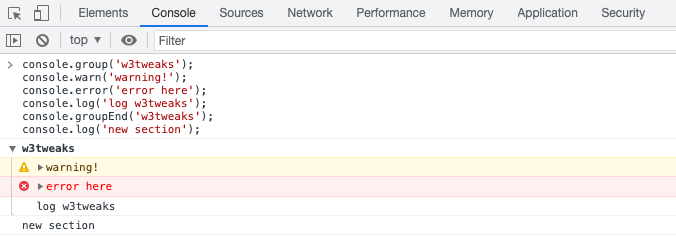
Leave a Reply